In this article, we will learn to create “Select Books” panel that displays the books of the selected category and subcategory. We
assume that the user has already selected category and subcategory of the book in the earlier panels. The information of the books will be fetched from the books
table in the server-side shopping
database. The list of the books matching the specified category and subcategory will be displayed as shown in figure 1(a). There can be multiple books in the subcategory, and these are
displayed sequentially. The user can scroll down to see additional entries, as shown in figure 1(b).
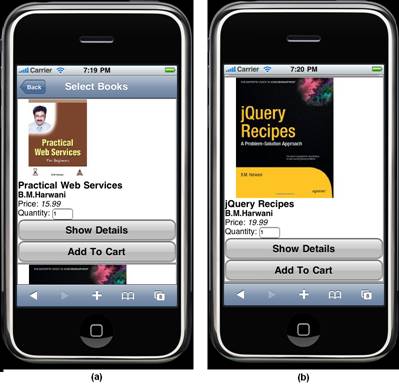
Figure 1 (a) List of books displayed in a selected subcategory (b) Scrolling the screen to view more books
To display all the books belonging to the selected subcategory, we need the following:
- A JavaScript function,
selectedsubcat()
, that manages the entire process A getbooks.php
PHP script that fetches books in the selected subcategory from the books
table- A
booksdisplay
div element that displays the books on the screen
Let’s first create the selectedsubcat()
JavaScript function, which will perform the following tasks:
- Calls the server-side
getbooks.php
PHP script, which fetches books belonging to the selected subcategory from the server-side books
database table. - Sends the books fetched by the PHP script to the
booksdisplay
div. - Jumps to the
booksdisplay
div, which outputs the book list to the screen.
The selectedsubcat()
code is shown in Listing 1.
Listing 1. selectedsubcat() function
function selectedsubcat(cat, subcat){
$('#bookslist').children().remove();
$.ajax({
type:"POST",
url:"getbooks.php",
data: 'category='+cat+'&subcategory='+subcat,
success:function(html){
$('#bookslist').append(html);
jQT.goTo('#booksdisplay', 'slide');
}
});
return false;
}
The sequence of events is as follows:
- The
cat
and subcat
parameters are passed to the selectedsubcat()
function that contains the category and subcategory selected by the user.
- Any of the books in a previously selected category and subcategory are removed from the
bookslist
div. - The
ajax()
method is called, requesting the POST method and the getbooks.php
server-side script be used.
- The selected category and subcategory is passed to the script in the variables
category
and subcategory
as data.
- The script file,
getbooks.php,
fetches
the books from the server-side books
database table that meet the criteria supplied by data.
- When the request sent to
getbooks.php
succeeds, the success
call back function executes. - The script response is received in an
html
parameter that is appended to the bookslist
div. - The
bookslist
div
is nested inside the booksdisplay
div,
hence we are
navigated to it via a slide animation and displays the list of books. - To suppress the browser’s default click-event behavior, the function returns
false
.
The code for the server-side PHP script, getbooks.php,
is shown in Listing 2.
Listing 2. getbooks.php script
<?php
$cat =trim($_REQUEST['category']);
$subcat =trim($_REQUEST['subcategory']);
$connect=mysql_connect("localhost","root",
"mce") or die ("Please check your server
connection");
mysql_select_db("shopping");
$query="Select isbn, title, author1, author2, author3,
price, image from books
where category =\"$cat\" and subcategory = \"$subcat\"";
$results =mysql_query($query) or die (mysql_query());
if(mysql_num_rows($results)>0)
{
while ($row=mysql_fetch_array($results))
{
extract ($row);
echo '<fieldset
style="background-color:white; color:black;">';
echo '<form action="cart.php?isbn=' . $isbn .
'&title=' .
urlencode($title) . '&price=' . $price
.'&action=add' . '"
method="POST"
class="form">';
echo '<img src=' . $image .'>';
echo '<h3>' . $title . '</h3>';
echo '<h4>' . $author1 . '</h4>';
echo '<label>Price: </label>';
echo '<em>' . $price .
'</em><br/>';
echo '<label>Quantity: </label><input
type="text" name="quantity"
value="1" style="height:22px;"
size="6"/>';
echo '<a class="whiteButton"
href="#" onclick="showdetails(\'' . $isbn .
'\');"> Show Details</a>';
echo '<a class="submit whiteButton"
href="#"> Add To Cart</a>';
echo '</form>';
echo '</fieldset>';
}
}
else
{
echo '<ul class="rounded">';
echo "<li> No Books found in this
Subcategory</li>";
echo "</ul>";
}
?>
The sequence of events is as follows:
- The category and subcategory selected by the user is passed to the script
getbooks.php
via data
. - The category and subcategory are retrieved from a
$_POST
array and stored in the $cat
and $subcat
variables. - A connection to the
shopping
database is established and a query executed to fetch information from the books
table that match the category and subcategory specified in the $cat
and $subcat
variables
respectively. - The list of books belonging to the specified category and subcategory is fetched from the
books
table and stored in the results
array.
- If the
$results
array is empty, that is, if there are no books of the selected category and subcategory, then a No Books found in this Subcategory
response is generated and sent back to the selectedsubcat()
function for display. - If the
results
array is not empty, rows are sequentially retrieved and assigned to the $row
variable. - The row retrieved in
$row
variable is extracted to display data stored in the image, title, author1,
and price
columns.
- A
quantity
input text field and two buttons, Show Details
and Add To Cart,
are attached to each book displayed. - The input text field
quantity
is assigned the default value of 1.
The user can change this value if desired.
- The
Add To Cart
button invokes the cart.php
PHP script file, which adds the selected book to the cart. - The
Show Details
button invokes the showdetails()
function. The selected book’s isbn
is passed to showdetails()
as a parameter. We will see in the next section how the showdetails()
function is used for displaying the selected book’s detailed information. - The response of the
getbooks.php
script is sent to the selectedsubcat()
function, which appends the response to the bookslist
div for displaying in the panel. The code for booksdisplay div
is shown in Listing 3.
Listing 3. booksdisplay div
<div id="booksdisplay">
<div class="toolbar">
<a class="back"
href="#">Back</a>
<h1>Select Books</h1>
</div>
<div id="bookslist">
</div>
</div>
The sequence of event is as follows:
- The
booksdisplay
div contains a nested toolbar
div that displays a Back
button for jumping to the Subcategories
panel. - The toolbar also contains a
Heading 1
element that displays the Select Books
title on the panel. Below the toolbar is a bookslist
div element. - The response generated by
getbooks.php
, containing all the books in the selected category and subcategory, is appended to bookslist
via the selectedsubcat()
function
B.M.Harwani is founder and owner of Microchip Computer Education (MCE), based in Ajmer, India that provides computer education in all programming and web developing platforms. He graduated with a BE in computer engineering from the University of Pune, and also has a 'C' Level (master's diploma in computer technology) from DOEACC, Government Of India. Being involved in teaching field for over 16 years, he has developed the art of explaining even the most complicated topics in a straight forward and easily understandable fashion. He has written several books on various subjects that includes JSP, JSF, EJB, PHP, .Net, Joomla, jQuery and Smartphones. To know more, visit http://bmharwani.com/blog