Problem
How to implement Azure AD B2C authentication in ASP.NET Core 2.0.
Solution
In previous posts, we have seen how to secure our applications using:
In this post, I’ll demonstrate how to use Azure AD B2C to delegate identity and access management to Azure. One of the key differences is that we will not pre-register users in Azure AD using Azure AD domain name, like previous post, instead consumers of our applications can create users using any domain, e.g., gmail.com.
Configure Azure AD B2C
Create Azure subscription (start for free, gives you credit to play).
Create new resource and search for ‘azure active directory b2c
’:
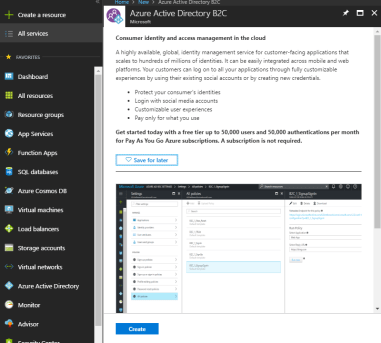
We’ll setup a new directory:

Enter details, including the unique ‘initial domain name’, this will become the Tenant Name that will be used in your application, e.g., below the tenant name will be fiveradb2c.onmicrosoft.com
:

Once directory is created, we need to register our application:

Once the application is created, you’ll see ‘Application ID’ in the list. This will be used in our application later as ‘Client ID’. Make a note of this.
Click on application and ‘Properties’, enter Reply URL where Azure AD will redirect user after sign-in, sign-out, sign-up and edit profile:

Note that these URLs are used by the middleware and match the ones shown above.
Next, we’ll setup policies for sign-in, sign-up and edit profile:

Here, I’ll show one policy (sign-up or sign-in) as all of them are very similar. First, we’ll setup identity provider:

Next properties/attributes that user will fill during sign-up:

Next claims that will be returned to your application:

Once you’ve setup all the policies, you’ll see them under ‘All Policies’. Note that Azure has prepended them with ‘B2C_1_
’:

So far you have:
- Created Azure AD B2C
- Registered your application and reply URLs
- Registered policies for sign-in, sign-up and profile editing
Next, we’ll setup our application and use Azure AD B2C to register and authenticate users.
Configure Application
Create an empty project and update Startup
to configure services and middleware for MVC and Authentication:
private readonly string TenantName = "";
private readonly string ClientId = "";
public void ConfigureServices(IServiceCollection services)
{
var signUpPolicy = "B2C_1_sign_up";
var signInPolicy = "B2C_1_sign_in";
var signUpInPolicy = "B2C_1_sign_up_in";
var editProfilePolicy = "B2C_1_edit_profile";
services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme =
CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultSignInScheme =
CookieAuthenticationDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = signUpInPolicy;
})
.AddOpenIdConnect(signUpPolicy, GetOpenIdConnectOptions(signUpPolicy))
.AddOpenIdConnect(signInPolicy, GetOpenIdConnectOptions(signInPolicy))
.AddOpenIdConnect(signUpInPolicy, GetOpenIdConnectOptions(signUpInPolicy))
.AddOpenIdConnect(editProfilePolicy,
GetOpenIdConnectOptions(editProfilePolicy))
.AddCookie();
services.AddMvc();
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseAuthentication();
app.UseMvcWithDefaultRoute();
}
Note that we have to add middleware to handle each policy setup on Azure AD B2C, to avoid repetition, I’ve added GetOpenIdConnectOptions
method:
private Action<OpenIdConnectOptions> GetOpenIdConnectOptions(string policy)
=> options =>
{
options.MetadataAddress =
"https://login.microsoftonline.com/" + this.TenantName +
"/v2.0/.well-known/openid-configuration?p=" + policy;
options.ClientId = this.ClientId;
options.ResponseType = OpenIdConnectResponseType.IdToken;
options.CallbackPath = "/signin/" + policy;
options.SignedOutCallbackPath = "/signout/" + policy;
options.SignedOutRedirectUri = "/";
};
Here, we are setting up Open ID Connect authentication middleware with:
MetadataAddress
: path to the discovery endpoint to get metadata about our policy ClientId
: application identifier that Azure AD B2C provides for our application ResponseType
: value that determines authorization flow used and parameters returned from server. We are interested only in authorization token for now. CallbackPath
path where server will redirect after authentication. We don’t need to create this in our application, the middleware will handle this. SignedOutCallbackPath
: path where server will redirect after signing out. SignedOutRedirectUri
: path where application will redirect after signing out. This is path to our application home page, for instance
Also when configuring authentication middleware, we’ve specified sign-up or sign-in as challenge scheme. This is the middleware ASP.NET Core will use for users who have not been signed in. Azure AD B2C will present them with login page with option to sign-up too.
Once signed in, we want to store their identity in a cookie, instead of redirecting to authentication server for every request. For this reason, we’ve added cookie authentication middleware and are using that as default sign-in scheme. If the cookie is missing, users will be ‘challenged’ for their identity.
Add a controller to implement login, logout, sign-up and profile editing actions:
public class SecurityController : Controller
{
public IActionResult Login()
{
return Challenge(
new AuthenticationProperties { RedirectUri = "/" }, "B2C_1_sign_in");
}
[HttpPost]
public async Task Logout()
{
await HttpContext.SignOutAsync(
CookieAuthenticationDefaults.AuthenticationScheme);
var scheme = User.FindFirst("tfp").Value;
await HttpContext.SignOutAsync(scheme);
}
[Route("signup")]
public async Task<IActionResult> SignUp()
{
return Challenge(
new AuthenticationProperties { RedirectUri = "/" }, "B2C_1_sign_up");
}
[Route("editprofile")]
public IActionResult EditProfile()
{
return Challenge(
new AuthenticationProperties { RedirectUri = "/" },
"B2C_1_edit_profile");
}
}
When logging out, it is important to sign-out from authentication server (Azure AD B2C) and also remove the cookie by signing out from cookie authentication scheme. Azure AD B2C sends the current profile name as tfp
claim type, we use that to sign-out from the policy currently in use.
Run the application:

Sign-up a new user:

Then login:

You’ll be redirected to your application:

You could edit the profile too:

When cancelling profile edit (or sign-up), Azure AD redirects back to your application but with an error, in order to catch that error and redirect to your home page we can handle event when setting up Open ID Connect middleware:
private Action<OpenIdConnectOptions> GetOpenIdConnectOptions(string policy)
=> options =>
{
...
options.Events.OnMessageReceived = context =>
{
if (!string.IsNullOrEmpty(context.ProtocolMessage.Error) &&
!string.IsNullOrEmpty(context.ProtocolMessage.ErrorDescription) &&
context.ProtocolMessage.ErrorDescription.StartsWith("AADB2C90091"))
{
context.Response.Redirect("/");
context.HandleResponse();
}
return Task.FromResult(0);
};
};