Download ComboBox.zip now!
Introduction
The component was developed to solve the problem when we have to use a text box while providing pre-defined options on a form.
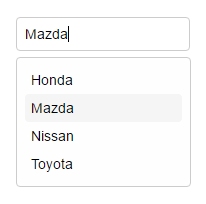
Using the code
The trick was to join a combo built with a "ul" list with a text box, using CSS to fit them and a bit of JavaScript to do the rest of the job.
HTML:
<div class="container">
<div class="comboBox">
<div class="comboGroup">
<input type="text" id="carBrand" placeholder="Select or type a new option..." />
<ul>
<li>Honda</li>
<li>Mazda</li>
<li>Nissan</li>
<li>Toyota</li>
</ul>
</div>
<script>new comboBox('carBrand');</script>
</div>
</div>
CSS:
<style>
.container {
padding: 50px;
}
*:focus {
outline: none;
}
.comboBox {
width: 400px;
}
.comboGroup {
position: relative;
width: 100%;
text-align: left;
}
.comboGroup * {
font-family: arial, helvetica, sans-serif;
font-size: 14px;
}
.comboGroup input {
padding: 8px;
margin: 0;
border: 1px solid #ccc;
border-radius: 4px;
width: 100%;
background-color: #fff;
z-index: 0;
}
.comboGroup ul {
padding: 8px 20px 8px 8px;
margin: 0;
border: 1px solid #ccc;
border-radius: 4px;
width: 100%;
background-color: #fefefe;
z-index: 1;
position: absolute;
top: 40px;
display: none;
}
.comboGroup li {
padding: 6px;
margin: 0;
border-radius: 4px;
width: 100%;
display: block;
}
.comboGroup li:hover {
cursor: pointer;
background-color: #f5f5f5;
}
</style>
Javascript:
<script>
function comboBox(id) {
var box = this;
box.txt = document.getElementById(id);
box.hasfocus = false;
box.opt = -1;
box.ul = box.txt.nextSibling;
while (box.ul.nodeType == 3) box.ul = box.ul.nextSibling;
box.ul.onmouseover = function () {
box.ul.className = '';
};
box.ul.onmouseout = function () {
box.ul.className = 'focused';
if (!box.hasfocus) box.ul.style.display = 'none';
};
box.list = box.ul.getElementsByTagName('li');
for (var i = box.list.length - 1; i >= 0; i--) {
box.list[i].onclick = function () {
box.txt.value = this.firstChild.data;
}
}
box.txt.onfocus = function () {
box.ul.style.display = 'block';
box.ul.className = 'focused';
box.hasfocus = true;
box.opt = -1;
};
box.txt.onblur = function () {
box.ul.className = '';
box.hasfocus = false;
};
box.txt.onkeyup = function (e) {
box.ul.style.display = 'none';
var k = (e) ? e.keyCode : event.keyCode;
if (k == 40 || k == 13) {
if (box.opt == box.list.length - 1) {
box.opt = -1;
}
box.txt.value = box.list[++box.opt].firstChild.data;
} else if (k == 38 && box.opt > 0) {
box.txt.value = box.list[--box.opt].firstChild.data;
}
return false;
};
box.ul.onclick = function (e) {
box.ul.style.display = 'none';
return false;
};
}
</script>
Points of Interest
It is very simple to use component to any HTML form! No Bootstrap or jQuery dependencies.
History
No updates yet.